Occasionally there may be some important alerts that you want your trading algorithms to send to you that require immediate attention. One that I use frequently is daily account drawdown. Here’s how you can set up Twilio to send you a text message when your account enters a certain percentage of drawdown.
Head over to Twilio
You do need to purchase a number to text from. It costs $1/month. You can find that here.
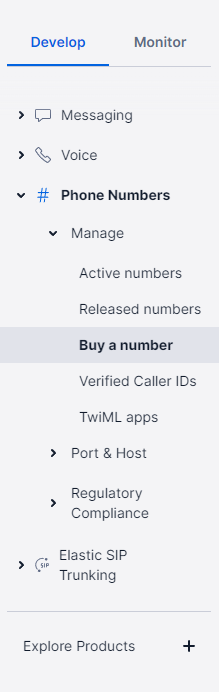
You’ll now need to go to your general settings page to retrieve your live credentials.
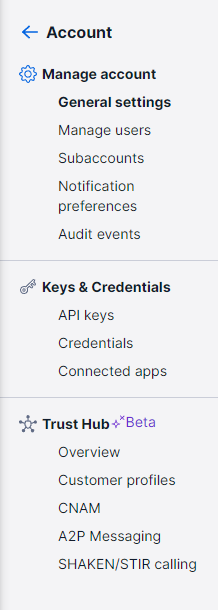
The next step is to install Twilio on your operating system. I use Ubuntu this can be done with the following command
sudo pip3 install twilio

Great now you’re ready to code.
The first thing you want to do is write a simple Python script to test your message sending capabilities. In the below code you’ll want to change a few variables:
account_sid – Change with your Twilio information found on your account page.
Auth_token – Change with your Twilio information found on your account page.
Using the format +19998887777 change to_num to your cell
Using the format +19998887777 change from_num to your Twilio phone number
def send_sms(to_num,from_num,body_message):
import os
from twilio.rest import Client
account_sid = 'Enter your account sid here'
auth_token = 'Enter your account token here'
client = Client(account_sid, auth_token)
try:
message = client.messages.create(
to=to_num,
from_=from_num,
body=body_message
)
print(message.sid)
except Exception as e:
print(e)
if __name__ == '__main__':
send_sms(+14809999999,+17149999999,'test')
Now that we have a function that can send text messages we just need to incorporate this into our code.