This is a simple tutorial on how to get started with TWS API from Interactive Brokers and subscribe to a few test symbols to get stock updates in Ubuntu.
You need to first install the Trader Workstation client – https://www.interactivebrokers.com/en/trading/tws-updateable-latest.php
wget https://download2.interactivebrokers.com/installers/tws/latest/tws-latest-linux-x64.sh
./tws-latest-linux-x64.sh
You need to launch the client and login with your credentials. You then need to go to Global Configuration and “Enable ActiveX and Socket Clients”
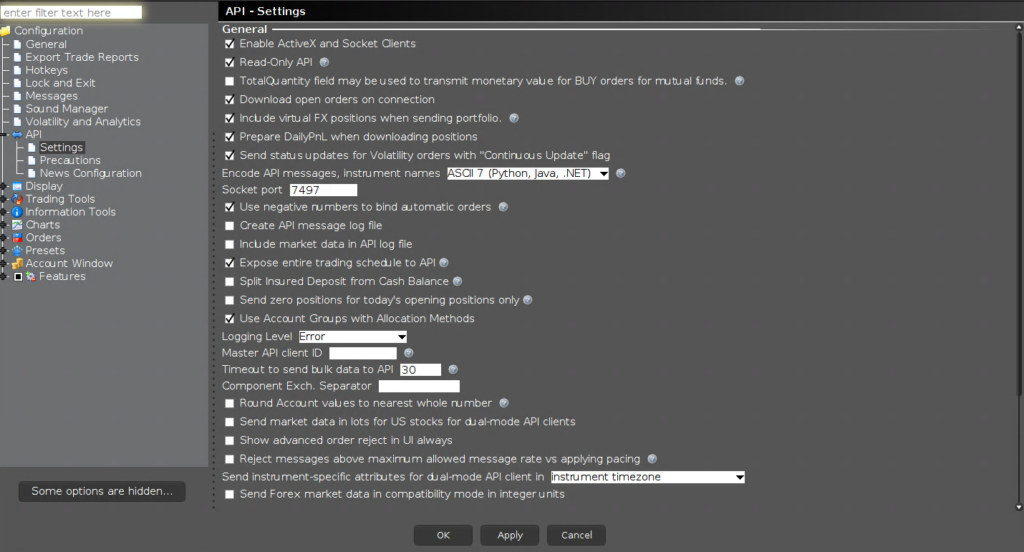
Next you need to download install the TWS API
Download and Unzip
wget https://interactivebrokers.github.io/downloads/twsapi_macunix.1019.01.zip
unzip twsapi_macunix.1019.01.zip
Install
cd IBJts/source/pythonclient
conda activate interacivebrokers
python3 setup.py install
Now that both of these are installed here is the Python code to subscribe to some data.
from ibapi.wrapper import EWrapper
from ibapi.client import EClient
from ibapi.contract import Contract
from ibapi.ticktype import TickTypeEnum
from threading import Thread
class IBQuotePrint(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
def error(self, reqId, errorCode, errorString, errorExtraInfo):
print(f"Error {reqId}: {errorCode} - {errorString}")
def tickPrice(self, reqId, tickType, price, attrib):
print(f"Request {reqId}: {TickTypeEnum.to_str(tickType)} - Price: {price}")
def main():
# Define the symbols you want to get quotes for
symbols = ['AAPL', 'GOOGL', 'MSFT']
# Create the IB client and connect to the API
app = IBQuotePrint()
app.connect('127.0.0.1', 7497, clientId=1)
# Start the message thread
thread = Thread(target=app.run)
thread.start()
# Define a function to create a stock contract
def create_stock_contract(symbol):
contract = Contract()
contract.symbol = symbol
contract.secType = 'STK'
contract.exchange = 'SMART'
contract.currency = 'USD'
return contract
# Request quotes for each symbol
for i, symbol in enumerate(symbols, start=1):
contract = create_stock_contract(symbol)
app.reqMktData(i, contract, '', False, True, [])
# Wait for user to press Enter to exit
input("\nPress Enter to exit...\n")
# Disconnect and clean up
app.disconnect()
thread.join()
if __name__ == "__main__":
main()