Here is a quick tutorial on how to generate API Key, Secret, and Passphrase to Polymarket.com. This way you can start trading using Python and skip the web interface.
keys.env
The first step is to create an environment file. You only want to populate the PK value. You do not know the other 3 variables yet so just comment them out. You can find this private key by logging in to your Polymarket.com account. Clicking on ‘Cash’, 3 dots, and finally Export Private Key.
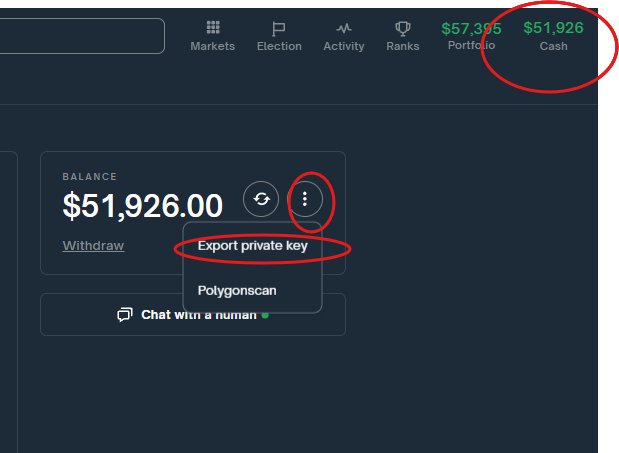
#remove the '0x' PK = "PRIVATE KEY EXPORTED FROM POLYMARKET.COM" #these are generated through get_api_key.py do not specify these until you run the script with your private key #API Key: xxxxxxxx-xxxx-xxxxx-xxxx-xxxxxxxxxxxx #Secret: Lxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx= #Passphrase: dxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxe9
generate_api_key.py
import os from py_clob_client.client import ClobClient from dotenv import load_dotenv # Load the environment variables from the specified .env file load_dotenv('keys.env') def main(): host = "https://clob.polymarket.com" key = os.getenv("PK") chain_id = 137 # Polygon Mainnet chain ID # Ensure the private key is loaded correctly if not key: raise ValueError("Private key not found. Please set PK in the environment variables.") # Initialize the client with your private key client = ClobClient(host, key=key, chain_id=chain_id) # Create or derive API credentials (this is where the API key, secret, and passphrase are generated) try: api_creds = client.create_or_derive_api_creds() print("API Key:", api_creds.api_key) print("Secret:", api_creds.api_secret) print("Passphrase:", api_creds.api_passphrase) # You should now save these securely (e.g., store them in your .env file) except Exception as e: print("Error creating or deriving API credentials:", e) if __name__ == "__main__": main()